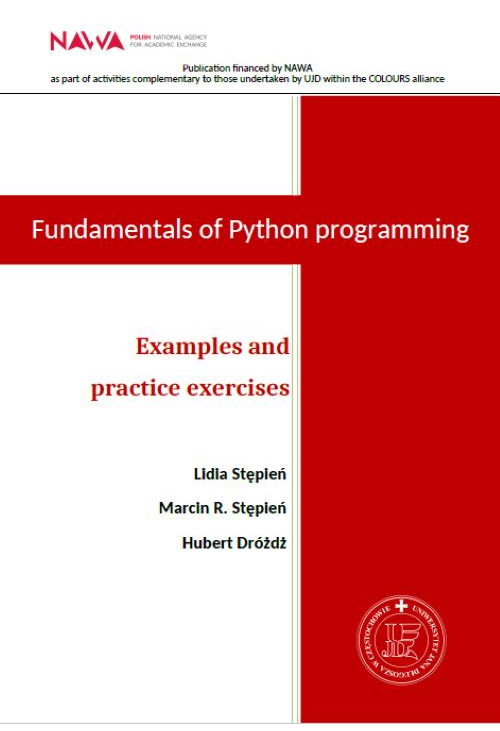
- Za darmo
ebook Fundamentals of Python programming. Examples and practice exercises
Lidia Stępień, Marcin R. Stępień, Hubert Dróżdż
Wydawca:
Uniwersytet Jana Długosza w Częstochowie
Rok wydania:
2024
Preface
A computer program is a detailed set of instructions that defines the actions that a computer
should perform. It is created as a result of the process of creating the program’s
source code in a selected programming language, which is a set of rules that determine
what sequences of symbols make up a computer program and what calculations describe
this program. At a later stage, the source code of the program can be processed through:
• compilation - the source code is translated into machine language,
• interpretation - the source code is continuously translated and executed by an additional
program called an interpreter.
Then we will say that the programming language subject to compilation is a compiled
programming language, and that subject to interpretation is an interpreted programming
language.
Python is an interpreted high-level programming language created by Dutch programmer
Guido van Rossum in 1990. The language was named after the BBC television program
"Monty Python’s Flying Circus". Python is developed as an Open Source project,
and its interpreters are available for various operating systems. Python is one of the
youngest, but also most commonly used programming languages today. It has a fairly
easy syntax, relatively few keywords, and a very rich library base, with the help of which
even very complex programming projects can be created. Python has rich possibilities for
both procedural and object-oriented programming. Another advantage is that the keywords
used in this language are identical to those in other modern high-level languages
such as C++, JAVA or PHP. However, compared to these languages, creating programs
in Python is more intuitive and does not require a beginner to have extensive computer
knowledge or remember many editing details. On the other hand, this language has some
interesting solutions that other languages do not have, including the existence of a computational
type for complex numbers, the exponentiation operator, a default input type of
str, a very convenient data structure list, and an essentially unlimited range of numeric
data.
Python is a multi-paradigm programming language with versatile applications, optimized
for code readability, concise syntax, and software quality. Python is currently
6
Preface 7
one of the most popular programming languages. It is used in various fields, from web
development and data analysis to artificial intelligence and machine learning. The large
community and wealth of libraries make Python ideal for both beginners and advanced
programmers.
Based on many years of teaching experience in teaching various programming languages
to first-cycle computer science students, and taking into account the varying levels
of programming advancement of students starting their studies, it can be stated with
certainty that learning Python as the first programming language seems to be the best
solution. Among other things, thanks to Python, students learn to take care of code readability
and it is easier for them to transfer these skills to other programming languages
that no longer have such restrictive requirements.
The proposed manual is a practical guide focusing on the basics of programming
in Python. Its primary goal is to develop programming skills in Python by discussing
basic programming constructs and data structures and providing rich illustrations using
appropriately selected examples. In addition, tasks for self-solving will serve to consolidate
the acquired skills. The reader will be able to use the skills acquired through studying
this manual in any Python-based software system encountered.
This manual could not have been created without the invaluable help of many people,
but dr hab. Andrzej Zbrzezny prof. UJD deserves special recognition. We could
always count on his support and suggestions resulting from many years of work with
students, as well as on inspiring us with his ideas. We are grateful for the opportunity to
draw from the resources of such rich scientific and teaching experience.
A computer program is a detailed set of instructions that defines the actions that a computer
should perform. It is created as a result of the process of creating the program’s
source code in a selected programming language, which is a set of rules that determine
what sequences of symbols make up a computer program and what calculations describe
this program. At a later stage, the source code of the program can be processed through:
• compilation - the source code is translated into machine language,
• interpretation - the source code is continuously translated and executed by an additional
program called an interpreter.
Then we will say that the programming language subject to compilation is a compiled
programming language, and that subject to interpretation is an interpreted programming
language.
Python is an interpreted high-level programming language created by Dutch programmer
Guido van Rossum in 1990. The language was named after the BBC television program
"Monty Python’s Flying Circus". Python is developed as an Open Source project,
and its interpreters are available for various operating systems. Python is one of the
youngest, but also most commonly used programming languages today. It has a fairly
easy syntax, relatively few keywords, and a very rich library base, with the help of which
even very complex programming projects can be created. Python has rich possibilities for
both procedural and object-oriented programming. Another advantage is that the keywords
used in this language are identical to those in other modern high-level languages
such as C++, JAVA or PHP. However, compared to these languages, creating programs
in Python is more intuitive and does not require a beginner to have extensive computer
knowledge or remember many editing details. On the other hand, this language has some
interesting solutions that other languages do not have, including the existence of a computational
type for complex numbers, the exponentiation operator, a default input type of
str, a very convenient data structure list, and an essentially unlimited range of numeric
data.
Python is a multi-paradigm programming language with versatile applications, optimized
for code readability, concise syntax, and software quality. Python is currently
6
Preface 7
one of the most popular programming languages. It is used in various fields, from web
development and data analysis to artificial intelligence and machine learning. The large
community and wealth of libraries make Python ideal for both beginners and advanced
programmers.
Based on many years of teaching experience in teaching various programming languages
to first-cycle computer science students, and taking into account the varying levels
of programming advancement of students starting their studies, it can be stated with
certainty that learning Python as the first programming language seems to be the best
solution. Among other things, thanks to Python, students learn to take care of code readability
and it is easier for them to transfer these skills to other programming languages
that no longer have such restrictive requirements.
The proposed manual is a practical guide focusing on the basics of programming
in Python. Its primary goal is to develop programming skills in Python by discussing
basic programming constructs and data structures and providing rich illustrations using
appropriately selected examples. In addition, tasks for self-solving will serve to consolidate
the acquired skills. The reader will be able to use the skills acquired through studying
this manual in any Python-based software system encountered.
This manual could not have been created without the invaluable help of many people,
but dr hab. Andrzej Zbrzezny prof. UJD deserves special recognition. We could
always count on his support and suggestions resulting from many years of work with
students, as well as on inspiring us with his ideas. We are grateful for the opportunity to
draw from the resources of such rich scientific and teaching experience.
Spis treści ebooka Fundamentals of Python programming. Examples and practice exercises
ContentsPreface 6
1 Introduction to Python 8
1.1 Installing Python 3 interpreter . . . . . . . . . . . . . . . . . . . . . . . . 8
1.2 Python language - the basics . . . . . . . . . . . . . . . . . . . . . . . . . 9
1.3 Working with interpreter . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15
1.4 Modules . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 18
1.5 Practice exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 22
2 Basic programming constructs 24
2.1 Conditional statement . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 24
2.1.1 Simple conditional statement . . . . . . . . . . . . . . . . . . . . . 24
2.1.2 Conditional statement with else clause . . . . . . . . . . . . . . . . 26
2.1.3 Complete conditional statement . . . . . . . . . . . . . . . . . . . . 27
2.2 Match case . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 29
2.3 Iteration statements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 31
2.3.1 while loop . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 32
2.3.2 for loop . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 36
2.4 Practice exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 40
2.4.1 Conditional statement . . . . . . . . . . . . . . . . . . . . . . . . . 40
2.4.2 Iteration instructions . . . . . . . . . . . . . . . . . . . . . . . . . . 43
3 Strings 46
3.1 Basic info . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 46
3.2 Indexes and substrings . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 49
3.3 Chosen str class methods . . . . . . . . . . . . . . . . . . . . . . . . . . . 51
3.4 String formatting . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 57
3.5 Practice exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 59
3
Contents 4
4 Functions 62
4.1 Function - general form . . . . . . . . . . . . . . . . . . . . . . . . . . . . 62
4.2 Function arguments . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 64
4.3 Function call . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 66
4.4 Practice exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 69
5 Data Structures 73
5.1 Lists . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 73
5.1.1 Lists - basic information . . . . . . . . . . . . . . . . . . . . . . . . 73
5.1.2 Chosen list class methods . . . . . . . . . . . . . . . . . . . . . . 77
5.1.3 Program call arguments . . . . . . . . . . . . . . . . . . . . . . . . 82
5.2 Tuples . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 84
5.3 Sets . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 87
5.3.1 Sets - the basics . . . . . . . . . . . . . . . . . . . . . . . . . . . . 87
5.3.2 Chosen set class methods . . . . . . . . . . . . . . . . . . . . . . . 90
5.3.3 Frozen sets . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 91
5.4 Dictionaries . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 92
5.4.1 Creating a dictionary . . . . . . . . . . . . . . . . . . . . . . . . . 92
5.4.2 Adding and overwriting data in a dictionary . . . . . . . . . . . . . 94
5.4.3 Deleting elements from a dictionary . . . . . . . . . . . . . . . . . 95
5.4.4 Dictionary inbuilt methods . . . . . . . . . . . . . . . . . . . . . . 96
5.4.5 Named function arguments . . . . . . . . . . . . . . . . . . . . . . 98
5.5 Practice exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 101
5.5.1 Lists . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 101
5.5.2 Sets . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 104
5.5.3 Dictionaries . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 105
5.5.4 Various tasks . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 106
6 Exception handling and working with files 107
6.1 Syntax errors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 107
6.2 Exception handling . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 108
6.3 Working with files . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 115
6.3.1 File opening modes . . . . . . . . . . . . . . . . . . . . . . . . . . . 116
6.3.2 Selected methods for text file objects . . . . . . . . . . . . . . . . . 117
6.3.3 Examples of working with text files . . . . . . . . . . . . . . . . . . 120
6.4 Binary files . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 123
6.4.1 Selected methods of binary file objects . . . . . . . . . . . . . . . . 128
6.5 Practice exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 130
Contents 5
7 Object-oriented programming 134
7.1 Basic concepts . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 134
7.1.1 Defining Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . 135
7.1.2 Encapsulating names in a class . . . . . . . . . . . . . . . . . . . . 137
7.2 Inheritance . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 140
7.3 Static and class methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . 144
7.4 Operator Overloading . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 147
7.4.1 Methods for comparing objects . . . . . . . . . . . . . . . . . . . . 148
7.4.2 Basic methods of binary operations . . . . . . . . . . . . . . . . . . 149
7.4.3 Right-side binary operations methods . . . . . . . . . . . . . . . . 150
7.4.4 Dual-argument methods with in place update . . . . . . . . . . . . 151
7.4.5 Other selected methods of action . . . . . . . . . . . . . . . . . . . 152
7.4.6 Example of class Vector . . . . . . . . . . . . . . . . . . . . . . . . 153
7.5 Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 156
7.6 Serializing Python Objects . . . . . . . . . . . . . . . . . . . . . . . . . . . 157
7.7 Practice exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 162
8 Advanced Python Elements 166
8.1 List comprehension . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 166
8.2 Anonymous functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 170
8.3 Enumerated types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 174
8.3.1 Class Enum . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 174
8.3.2 IntEnum class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 180
8.3.3 Flag Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 181
8.3.4 Class IntFlag . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 183
8.4 Iterators . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 184
8.4.1 Module itertools . . . . . . . . . . . . . . . . . . . . . . . . . . . 192
8.5 Generators . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 198
8.6 Practice exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 204
8.6.1 Iterators . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 204
8.6.2 Itertools module . . . . . . . . . . . . . . . . . . . . . . . . . . . . 205
8.6.3 Generator functions . . . . . . . . . . . . . . . . . . . . . . . . . . 206
8.6.4 Generator expressions . . . . . . . . . . . . . . . . . . . . . . . . . 206
8.6.5 Additional tasks . . . . . . . . . . . . . . . . . . . . . . . . . . . . 207
Bibliography 208
Szczegóły ebooka Fundamentals of Python programming. Examples and practice exercises
- Wydawca:
- Uniwersytet Jana Długosza w Częstochowie
- Rok wydania:
- 2024
- Typ publikacji:
- Ebook
- Język:
- angielski
- Format:
- ISBN:
- 978-83-679-8415-7
- ISBN wersji papierowej:
- 978-83-679-8415-7
- Autorzy:
- Lidia Stępień,Marcin R. Stępień,Hubert Dróżdż
- Miejsce wydania:
- Częstochowa
- Liczba Stron:
- 208
Recenzje ebooka Fundamentals of Python programming. Examples and practice exercises
-
Reviews (0)

Na jakich urządzeniach mogę czytać ebooki?
- Za darmo
0,00 zł

@CUSTOMER_NAME@
@COMMENT_TITLE@
@COMMENT_COMMENT@